Okay, so “the dance is deadly,” huh? Sounds dramatic, and honestly, it kinda was.
It all started last week. I was messing around with some Python scripts, trying to automate a really tedious data processing task. I figured, “Hey, I can write a script to handle this,” which is usually my first mistake. I started by sketching out the basic workflow, just a few steps: read the data, clean it up, do some calculations, and then spit out the results. Seemed simple enough.
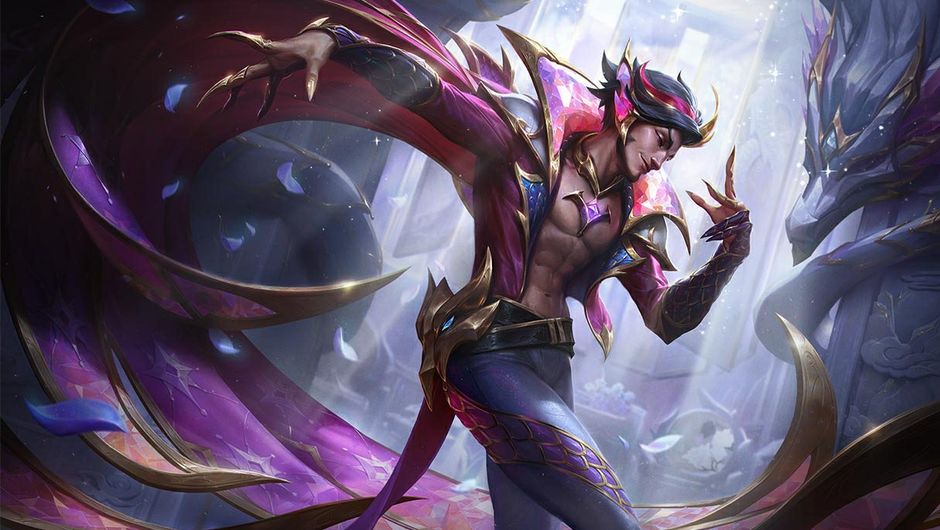
I fired up my editor and started banging out the code. First, the data reading part. I used Pandas, because who doesn’t? Wrote a little function to load the CSV, handle missing values, that sort of thing. Tested it, and it seemed to work fine. Okay, so far so good.
Then came the data cleaning. This is where things started to get messy. The data was, shall we say, less than pristine. Dates were in all sorts of formats, there were random typos in the categories, and don’t even get me started on the inconsistent units. So, I spent a good chunk of time writing regular expressions to normalize the dates, used a bunch of .replace()
calls to fix the typos, and added some unit conversion functions. It was a slog, but I eventually got it to a point where it was… acceptable.
Next up, the calculations. This was the heart of the whole thing, the reason I was automating it in the first place. I had to calculate some statistical metrics, like averages, standard deviations, and correlations. I used NumPy for this, which made it relatively straightforward. Wrote a few functions to do the calculations, and then tested them with some sample data. Everything seemed to check out.
Finally, the output. I wanted to generate a report with the results, so I decided to use a simple text file. Wrote a function to format the data nicely and then wrote it to a file. Done! Or so I thought.
I ran the script on the full dataset, and that’s when the dance began. It started crashing. Not consistently, mind you. Sometimes it would run for a few minutes, sometimes it would crash immediately. The error messages were cryptic and unhelpful. “IndexError,” “TypeError,” “KeyError” – it was like a greatest hits of Python exceptions.
I spent the next few hours debugging. I added print statements everywhere, tried different inputs, used the debugger to step through the code line by line. It was maddening. I realized that the errors were happening in different places each time, which suggested some kind of race condition or memory issue. I suspected it was related to the way I was handling the data in Pandas, so I started experimenting with different approaches.
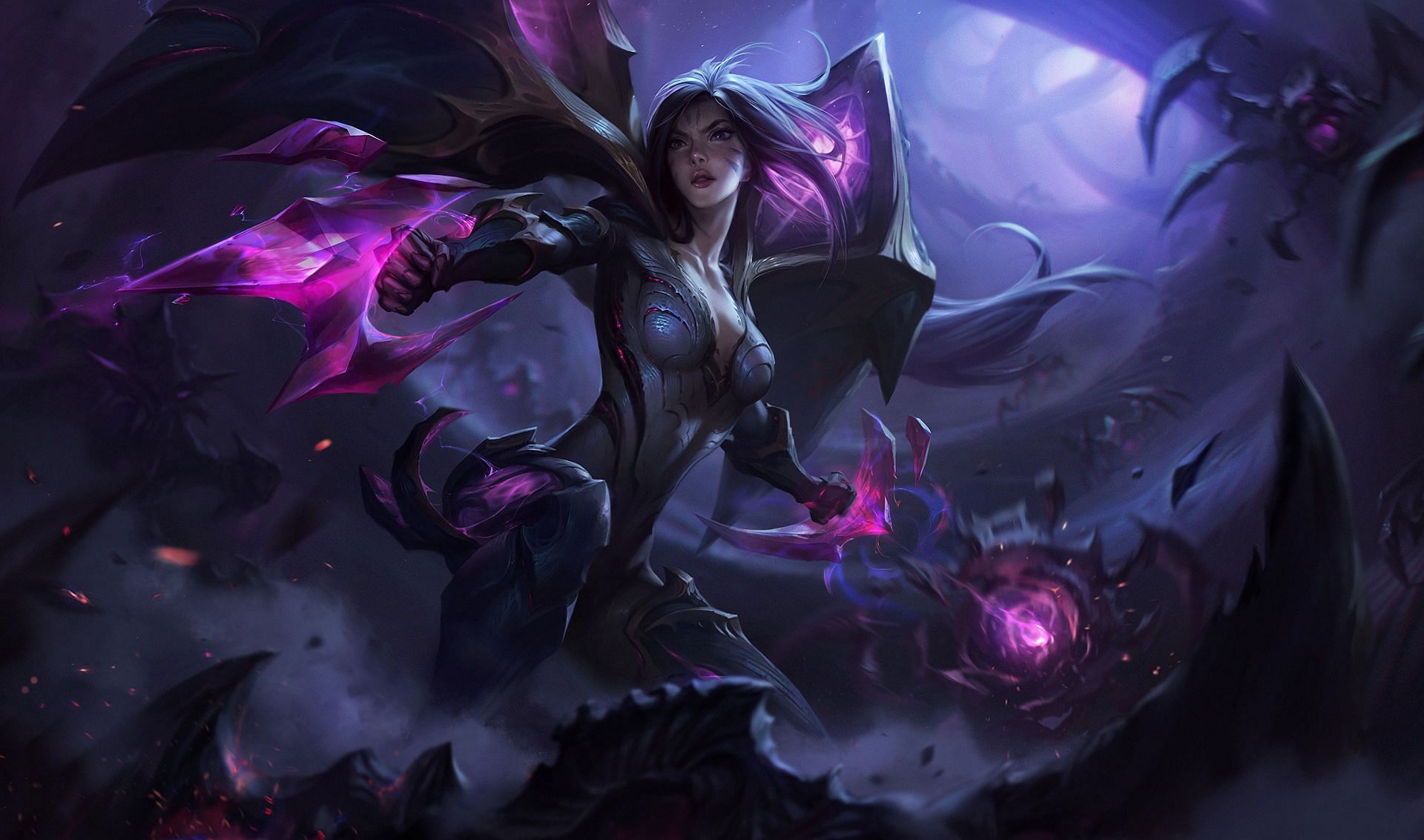
Eventually, after much trial and error, I discovered the problem. It turned out that some of the data had unexpected characters that were causing Pandas to choke. I added a step to remove those characters before processing the data, and suddenly everything started working. The script ran smoothly, the results were accurate, and I finally had my automated report.
So, “the dance is deadly” refers to the debugging waltz I had to perform, a seemingly endless back-and-forth between error messages, code tweaks, and re-runs. But hey, I learned a lot in the process. The key takeaway? Always, always, always sanitize your data. You never know what kind of weirdness lurks in the shadows. And sometimes, a simple print statement can be your best friend.