Okay, so today I wanted to mess around with preprocessing some data for, like, a game or something. I had this idea for handling “party members” – you know, the characters that team up with the main player. The title I came up with was “party member preprocessing apex”. I just wanted to try and make it all smooth and efficient in Apex.
Getting Started
First, I created a new Apex class. I called it `PartyMemberPreprocessor`. I didn’t have any data to start with so it was pretty barebones, just a simple class declaration.
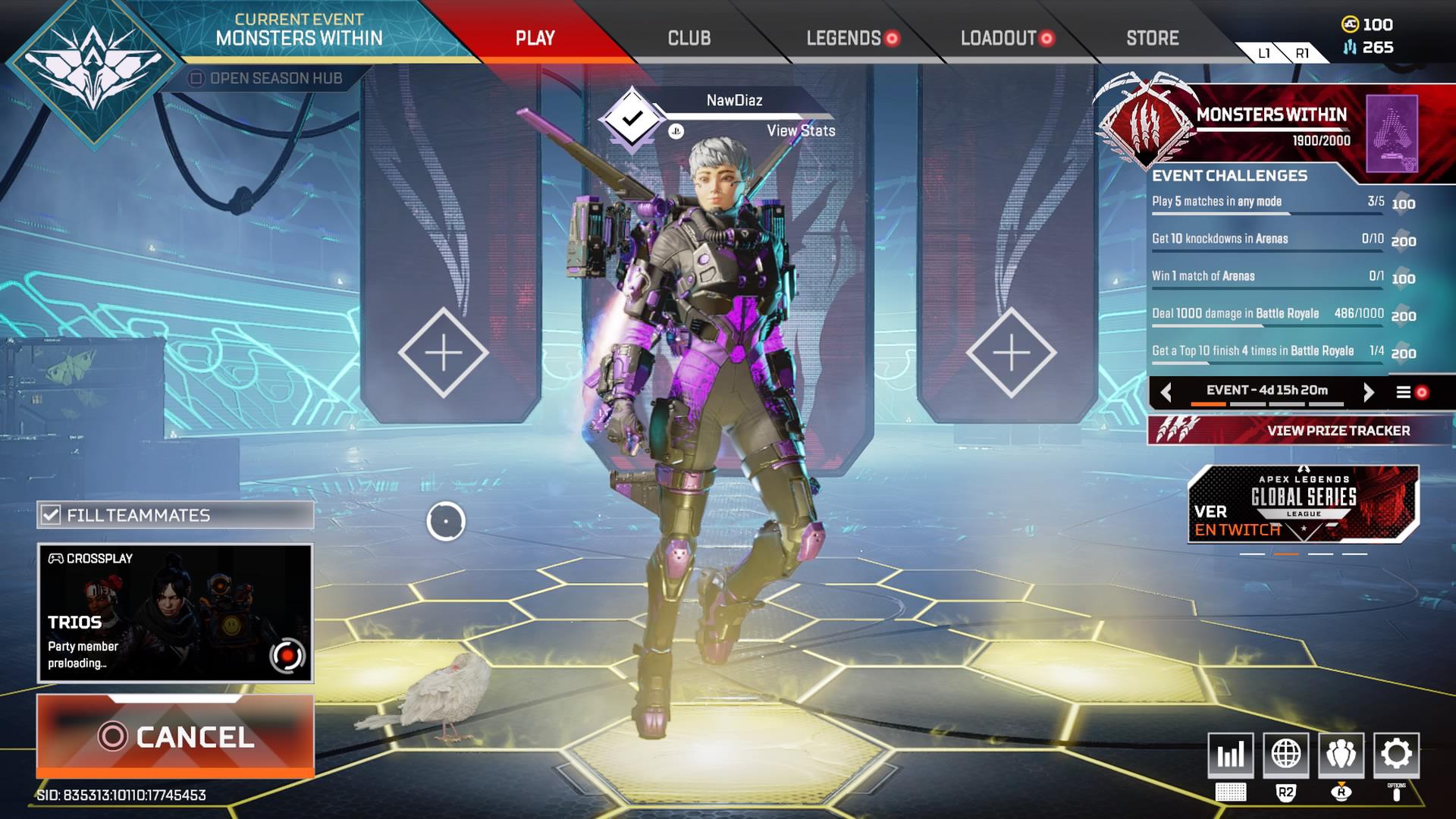
Thinking Through the Data
I started to think about what kind of data I’d actually need. Stuff like, I don’t know:
- Name (obviously!)
- Some kind of ID
- Maybe their level?
- Stats? Like, strength, speed, and that kind of thing.
- What skills or abilities they have.
I figured I could probably represent all this with a custom Apex class, maybe call it `PartyMember`. So I made another class to represent it. Inside it, I added some simple properties with the appropriate data types, I think it was String, Integer, and so on.
Building the Preprocessing Logic
Back in my `PartyMemberPreprocessor` class, I wrote a method. Maybe I called it `preprocessPartyMembers` or something similar. The idea was to take in, say, a List of `PartyMember` records and do… something to them.
I wasn’t really sure what I wanted to do yet. I thought about:
- Maybe checking for invalid data? Like, if a level was negative, or a name was blank.
- Perhaps calculating some derived stats? Like, maybe a total power level based on their individual stats.
- Possibly even sorting them? Like, by level, or by name, or whatever.
I decided to start simple. I implemented the invalid data check first. I just looped through the list of party members and added some `if` statements to check for the basic stuff – negative levels, empty names, things like that.
Testing It Out
Of course, I needed to test this thing. I created a simple test class (`PartyMemberPreprocessorTest`… creative, I know). Inside, I added a test method and created a few `PartyMember` records, some with valid data, some with deliberately invalid data.
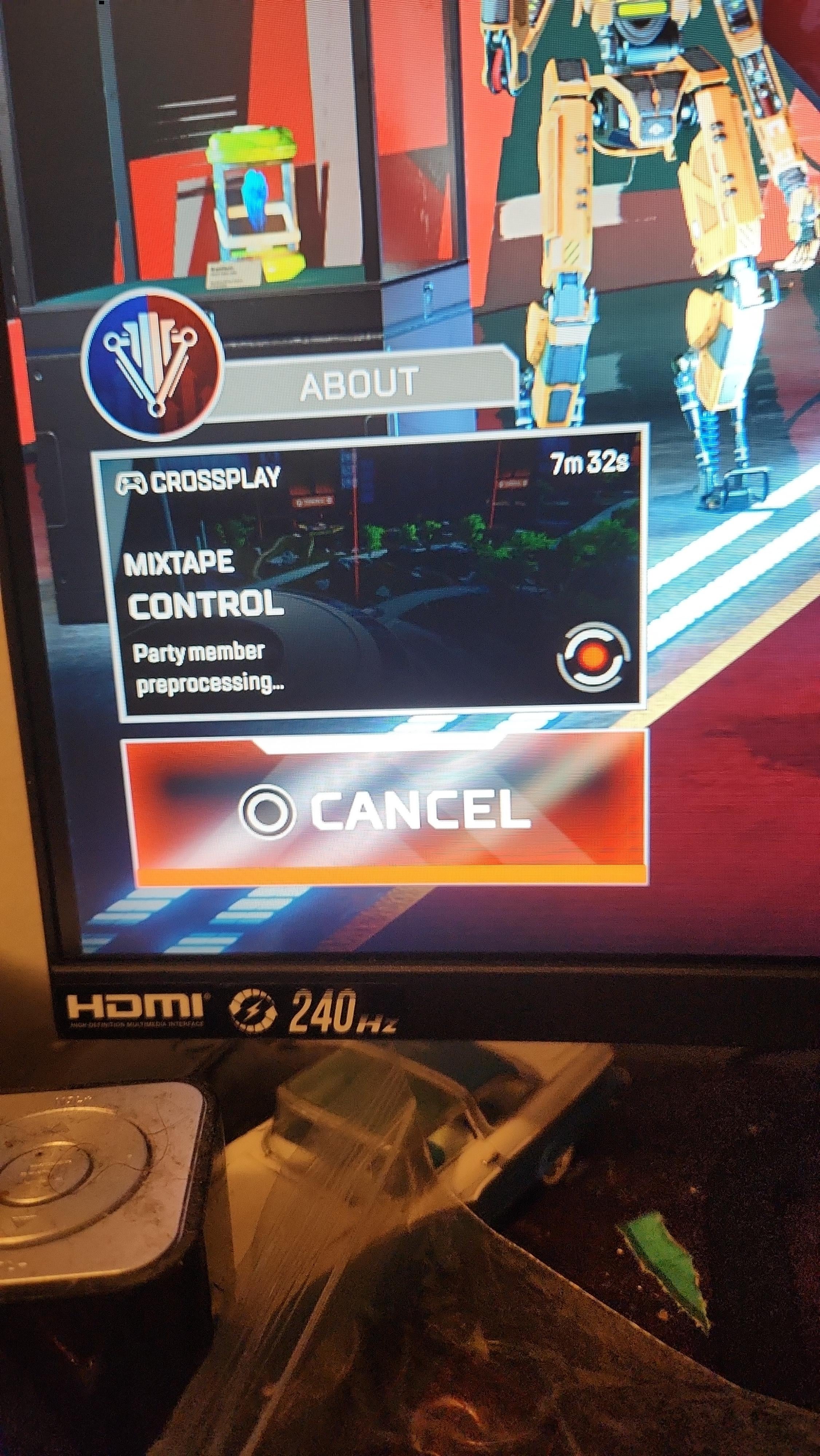
Then I called my `preprocessPartyMembers` method and used `*` statements to check the results. I wanted to make sure it was correctly flagging the bad data and leaving the good data alone.
Iterating and Improving
It didn’t work perfectly the first time, naturally. I found a few bugs, fixed them, and ran the tests again. You know, the usual debugging dance.
Once the basic validation was working, I added the derived stat calculation. That was a bit trickier, figuring out the formula I wanted to use, but I got it working eventually.
Finally I try the sorting. I used sort methods provided by the Apex to the list of member objects. And to show the final result, I added debug logs to print all data after processing.
And that’s it! The preprocessing work is done, I made those member data clean and ready to use!