Okay, so I’ve been seeing a lot of buzz about the Builder pattern lately, and honestly, I was a little skeptical. I mean, it seemed like extra work for something I could already do. But, I decided to give it a shot, a real hands-on test to see if this “Builder Apprentice” thing was worth my time. I’m calling it “Apprentice” because I’m literally learning this pattern.
My Little Experiment
I started simple. I didn’t jump into some massive project. Instead, I thought, “Let’s build a pizza.” Not a real pizza, obviously, a digital one. A `Pizza` class.
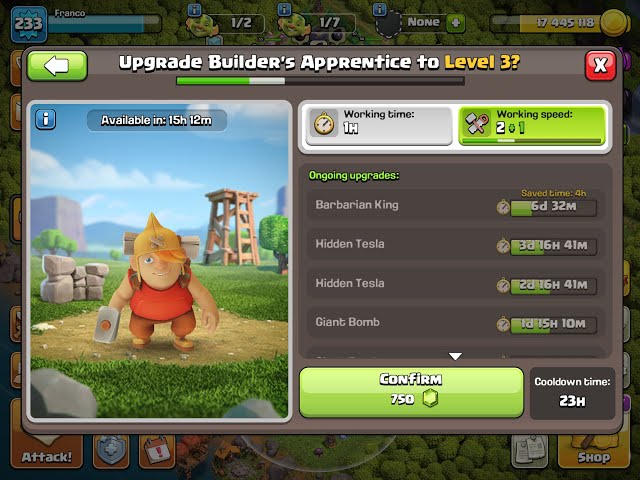
Normally, I’d just create a `Pizza` class with a constructor that takes a bunch of arguments: size, crust type, toppings, cheese, sauce… you get the idea. But with a builder, things are different.
First I created a `Pizza`class,only read-only properties inside.
Then, I crafted an inner class, my `PizzaBuilder`. This little guy had all the same fields as the `Pizza` class, but they were settable.
I Created methods like `withSize()`, `withCrust()`, `addTopping()`, and so on. Each of these methods would set the corresponding field in the builder and then – here’s the key – return the builder itself. This allows for that sweet, sweet method chaining.
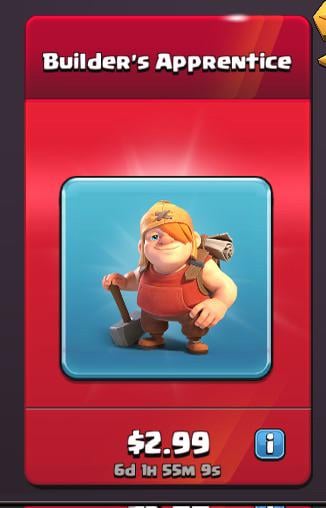
I added set methods like these.
- withSize(size): Set pizza’s * builder self.
- withCrust(crustType):Set pizza’s * builder self.
- addTopping(topping): Set pizza’s * builder self.
The `build()` method was the grand finale. It took all the settings from the builder and used them to create a brand new `Pizza` object. It’s like the builder hands over all the ingredients, and the `build()` method does the actual baking.
Chain, Chain, Chain…
The cool part? Using the builder. Instead of a giant constructor call, I could do something like this:
I tried to created a large, thin-crust pizza with pepperoni and mushrooms like this:
`*(“Large”).withCrust(“Thin”).addTopping(“Pepperoni”).addTopping(“Mushrooms”).build()`
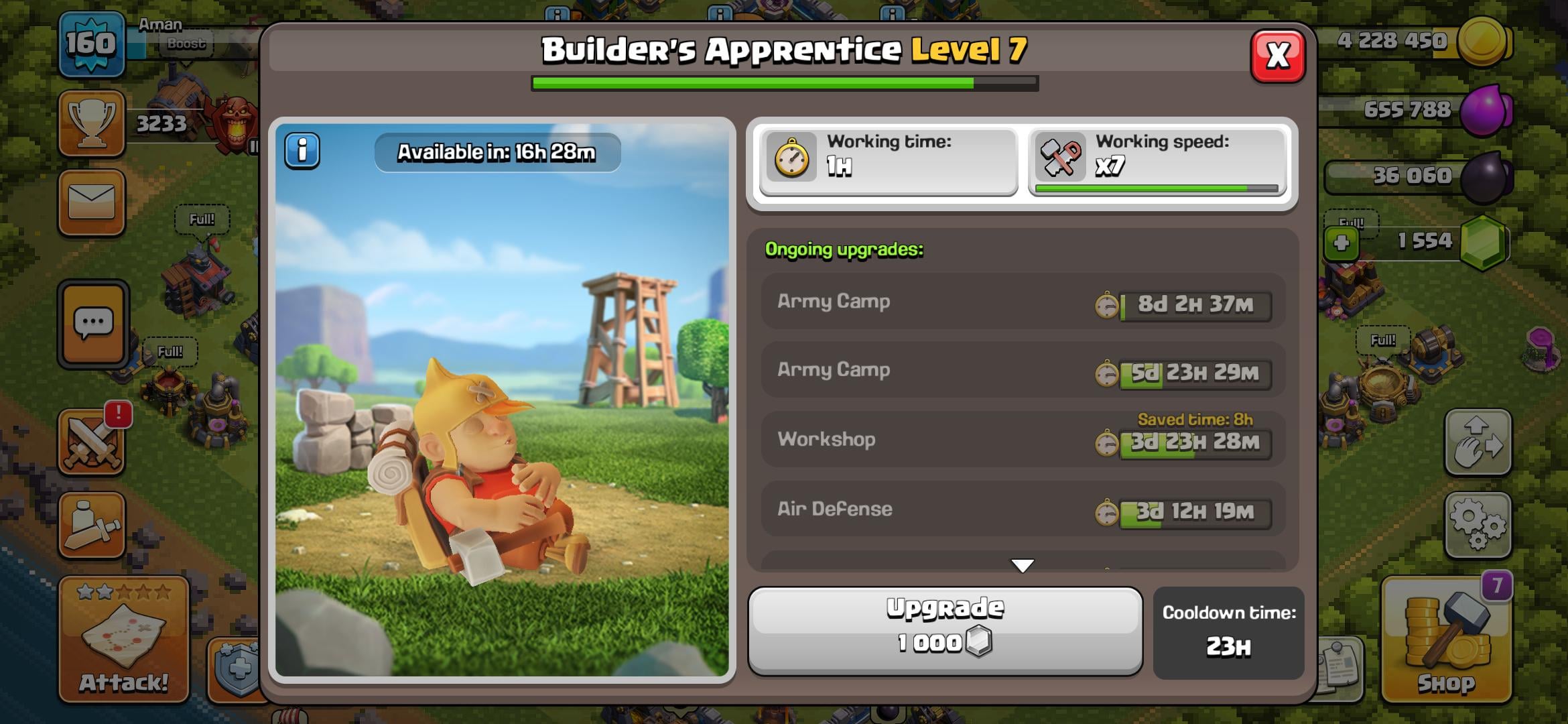
See that? It’s like building the pizza step-by-step. Each method call sets one thing, and then I chain on the next. It’s almost… * `build` action will return a `Pizza` instance for me.
The Verdict?
So, is the Builder Apprentice worth it? For my little pizza experiment, yeah, I think it *’s more code upfront, no doubt. I had to write that extra `PizzaBuilder` class. But the payoff is in readability and flexibility.
When I look back at this code later, I’ll be able to instantly understand how the pizza is being created. No more deciphering giant constructor calls.
If I want to add more options later – different sauces, cheeses, whatever – I can just add more methods to the builder. I don’t have to mess with the `Pizza` class itself, or worry about breaking existing code that uses the old constructor.
It felt a little weird at first, but after using it, I get the appeal. It’s like having a well-organized kitchen instead of just throwing all your ingredients into a pot and hoping for the *,my test is pass.I think I will use it in future.